Back to Basics: Software Syntax
February 14, 2024
Blog
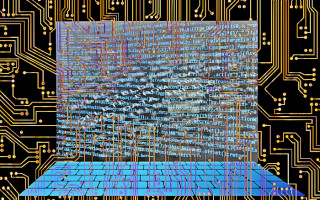
Up to this point in our series, we’ve intentionally kept things pretty broad. The fundamentals in the last two “levels” of software are relatively applicable across most languages, but now it’s time to get a bit more specific. And the first step to that is looking at the concept of software syntax.
Welcome to Back to Basics, a series where we’re going to be reviewing basic engineering concepts that may require a more complex explanation than a quick Google search could provide.
Syntax, as you may know, is actually a general language term that encompasses the rules you use to make a sentence out of words. In English, the sentence “The boy kicked the ball,” makes sense, while “Ball boy the kicked the,” does not. This is because we have syntactic rules that define the meaning behind how we construct sentences.
Notice that for this example, we had to use a specific language. We cannot say that there are syntactic rules for all languages, because, like it or not, all languages have different rules. There are, of course, similarities, but overall, when we look at the syntax of a language, we only look within the scope of the language itself.
To bring this back to software, every programming language has its own syntax. In Java, for example,
int x = 5; char myChar = ‘c’; String myWord = x+” and “+myChar;
makes sense, while
int x = 5 myChar = ‘c’ int Word = x+myChar;
does not, due to syntactic rules like variable typing and line terminators.
Common Syntax
Let’s look at a few common elements of syntax in programming that will give us a broader understanding of general language elements.
Typing
Some languages require that every time you make a variable, you give it a specific type. Remember how variables are like boxes? In a strongly typed language, rather than just saying, “I have a box” when you create a new one, you must say “I have a box that only holds integers, and nothing else.” There are advantages to both strongly typed and dynamically typed languages, as with typed languages, you don’t have to worry about whether you’re adding an integer to a word. “Happy” + 45 = bad news…
Indentation
Remember learning about indentation in your ELA classes? Well, in programming, indentation is sometimes more important. Often, in coding, indentation shows when one line of code is run inside of a control structure. Take a look at the C++ snippet below.
for(int i = 0; i<myNum; i++){ myNum = i + (i / myNum); }
The line “myNum = i + (i / myNum);” is indented inwards to show that it belongs inside of the “for” loop. In C++, indentation is optional, meaning that this
for(int i = 0; i<myNum; i++){ myNum = i + (i / myNum); }
or even this
for(int i=0;i<myNum;i++){myNum=i+(i/myNum);}
is just as valid as the previous example. They might look messier, but they would still work. But in some languages, like Python, indentation is required.
Line Terminators
In English writing, we end a sentence with a period, and to begin the next, we capitalize the first word. In programming, there is often a punctuation mark used to denote when a line of code is complete, but as is the case with any other syntactic structure, it’s not a universal rule.
One of the most common line terminators is the semicolon “;”. In Java, C, and C++, the semicolon is used at the end of every finished line of code. In Python, the semicolon is optional. Take a look at this Python code:
myNum = 5 while(myNum<25): myNum = myNum + 2
Notice the lack of typing on the variable “myNum,” the lack of semicolons, and the use of indentation. It’s easy to see where the end of each line of code is, because indentation is required, so the semicolon could be seen as superfluous.
There are plenty of other language-specific syntactic rules, but the above examples are just some of the basics. If you want to learn more, check out the additional resources below. In our next article, we’ll look at a few final common elements of software, like objects and functions!
ADDITIONAL RESOURCES
- What is Syntax? Definition, Rules, and Examples | Grammarly
- Difference Between Syntax and Semantics - GeeksforGeeks
- Role of SemiColon in various Programming Languages - GeeksforGeeks
- What is a strongly typed programming language? (techtarget.com)
- Java Cheat Sheet (2023): Freshers and Experienced
This is part three of a series on Software Language Essentials, as part of our regular Back to Basics Feature.
Part one: Software Language Essentials
Click here for more Back to Basics.