Back to Basics: Objects & Functions
February 21, 2024
Blog
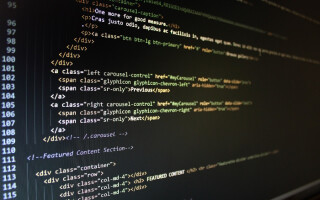
We’ve worked our way from machine code up to language-dependent syntax, and hopefully, if you’ve stuck with us, those two terms make sense! Now, we move on to two final general software structures: functions and objects.
Welcome to Back to Basics, a series where we’re going to be reviewing basic engineering concepts that may require a more complex explanation than a quick Google search could provide.
HOW FUNCTIONAL!
Functions are a common element of high level programming languages that allow developers to take a bunch of lines of code that do something and put them in a larger box. Think about how in a factory there are a bunch of different machines to produce a product. Each machine has an input and an output, and in the middle, it does something. Functions work exactly the same way. Let’s look at a basic C function:
float avgTwo(float x, float y){ return (x + y) / 2; }
This function, named “avgTwo,” averages two numbers together. In the first set of parentheses we see the input to the “machine.” In the input are two special variables called parameters. These are meant to be replaced with something else when the function is run.
At the end is the return statement, or output for your machine. The function returns the average of the two parameters, and then it ends. Simple, right?
This first bit of code is called a function declaration. It’s like the blueprint for our machine. When you want to actually use your function, you just need to call the name of the function, and the computer will look back at the blueprint to figure out what needs to be done.
Now, whenever you write code in this project and want to average two numbers, rather than writing out the entire formula, all you need to do is put
avgTwo(3,5);
and the work is done for you. With functions, you can create any machine you want, with as many inputs as you like, and one output, and it makes the coding process much simpler.
THERE’S ALWAYS A BIGGER BOX
By now, you might have noticed a pattern with programming. Every time we examine a higher level, there are more boxes to put things in. We started way back at the beginning with variables, which allow you to put basic information in boxes, and now we’ve moved up to functions, which allow you to put actions into bigger boxes.
Well, you’re not going to believe this… there are still bigger boxes.
Objects are the box to end all boxes. Found in object-oriented languages like Java, C++, and Python (sort of), objects can contain both functions and variables.
The easiest way to think about objects is to think of a car. Cars come in many different sizes, colors, and shapes, and they can do different things. Some cars have powered windows, others have automatic transmission, while others can self drive.
When an object is declared, you put all the information of what your “car” can be into special variables, and all the information of what our “car” can do goes into special functions. Here’s a Java example of a car object declaration:
public class Car{ int size; int width; int maxSpeed; String color; void driveForward(int distance){ //drives the car forward by “distance” } void driveBackward(int distance){ //drives the car backward by “distance” } void honkHorn(){ //honks the car’s horn } }
Just like with functions, this class declaration is the blueprint for anytime you make a new car in your code. When you make a new car, like this:
Car myCar = new Car();
you can then change any of its attributes or use any of its functions without having to write any new code. You just fill in the new traits you want the car to have:
myCar.size = 15; myCar.maxSpeed = 300; myCar.color = “Red”; myCar.honkHorn();
HIP HIP… ARRAY!
Some object-oriented programming languages come with built-in objects. A super common type of object would be an array. An array lets you take a type of variable or object and put a whole bunch of them in a line. When you’re programming, you’ll often have lists of ordered data, and arrays make the perfect box to store them in. Beyond just being a box like a variable, arrays as objects also contain class variables like the information of how many items are in the array.
Here’s a Java array of integers, for example:
int[] myInts = new int[]{1,2,3,4,5,6,7,8,9}; myInts.length;
When we call for the “length” class variable, we get the length of the array, which in this case, is 10.
One of the easiest ways to understand arrays is to look at strings, which are a special type of array. Strings are an array of characters, or single letters. When we look at a word, like “hello”, we can see it as an array of five characters: ‘h’,’e’,’l’,’l’,’o’.
With that, we’ve come to the end of our software vocab journey. From variables to syntax to objects, you should at least be able to understand the fundamentals of the crazy thing we call programming.
ADDITIONAL RESOURCES
This is part four of a series on Software Language Essentials, as part of our regular Back to Basics Feature.
Part one: Software Language Essentials
Click here for more Back to Basics.