Back to Basics: Get Coding and Commenting
September 13, 2023
Story
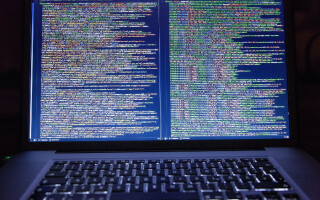
At this stage of your project, you’ve not only meticulously chosen and gathered materials, resources, tools, and an IDE that’s suitable for your project; but you’ve also created your pseudocode roadmap. And now, finally, it’s time to begin the real coding.
Welcome to Back to Basics, a series where we’re going to be reviewing basic engineering concepts that may require a more complex explanation than a quick Google search could provide.
Let’s Get Cooking!
Coding is the process of writing instructions in a programming language that, in most cases, a computer can’t fully read, but can be compiled into the binary language a CPU can understand.
It’s essential that you (and anyone else that may work with your code) can understand the instructions you create when programming. Software sits in a special space between what a human can understand and what a computer can process, but doesn’t fit fully into either category. As such, you need to provide something extra as you write your code to ensure that it’s human-readable: comments.
Annotating Isn’t Just for Books
It’s easy to dismiss code comments if you’re just thinking no one else will ever look at your code and you’ll never use it again. Those things may be true, but there’s no guarantee you won’t need that code in the future, when your recollection of how it functions has faded.
Good commenting is a balance between too much information and too little. If you’re doing something basic like initializing parameters or opening your main function, there’s no need to add a comment that says “creating and initializing parameters.” It’s basic enough that it speaks for itself. On the other hand, if you’re several hundred (or thousand) lines into a complex function, it’s easy to lose track of how it works if there are no comments.
A good rule of thumb is to rely on two different types of commenting: headers and clarifiers.
Headers are used when you start a function or a class, and they explain the name of the code block, what it does, and what inputs and outputs it contains.
/**
* This is a function that takes in an array of numbers and averages
* them.
* @param arr[] the array being averaged
* @return the average as a double
* @since 2.1
*/
public int averageNums(int[] arr){
}
In the example above, JavaDoc, a built-in standardized commenting system for Java, is used to show exactly what a function is supposed to do. This particular header comment quickly delineates the name, purpose, inputs and outputs, and versioning of the function “averageNums” so that anyone can quickly tell what the function does and how it works without having to read through all the code contained within the function.
Clarifier commenting is used when a simple header isn’t enough. Long blocks of code can tend to blend together, so when a thought or purpose in a block of code is complete, add a single-line comment in front to show what it does.
Where to Source Your Coding Queries
As you set off to begin your code, remember that getting stuck is normal. But before you panic and run your questions to Google or ChatGPT, take a beat. It’s important to know where the right (and wrong) places are to go for good answers. The internet contains a plethora of sources, not all of them reliable.
The first place to check for simple syntactic questions is always the code authors themselves. If you want to know how something works, the best authority is always the place of origin. If you’re looking at something more conceptual, there are great, credible teaching platforms that contain fact checked information on how to do things, including GeeksForGeeks and TutorialsPoint.
StackOverflow and ChatGPT may seem like fast, easy solutions to your questions, but there’s no guarantee they’ll work or even be correct. It’s always best to go with a known and reliable solution.
With those tips in your toolbelt, you’re set for a successful coding process. In the next part of this series we’ll tackle the darker half of programming: debugging. Until then, happy coding!
Additional Resources:
In the next part of this series we’ll tackle the darker half of programming: debugging. Until then, happy coding!
Click here to read the first installment of Back to Basics: Ideation & Requirements
Click here to read the second installment of Back to Basics: Development Stack
Click here to read the third installment of Back to Basics: Configuring the Development Environment
Click here to read the fourth installment of Back to Basics: Pseudo-coding